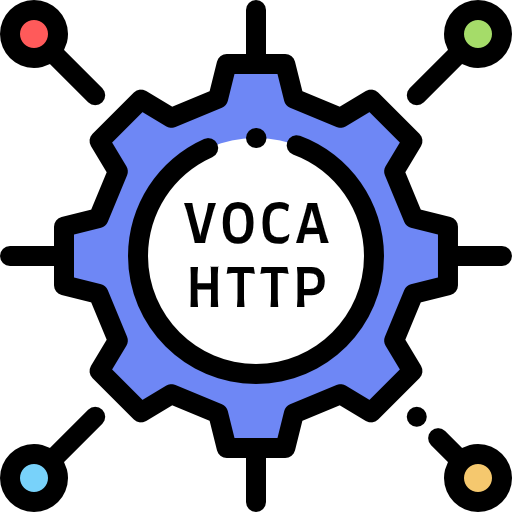
Voca HTTP
A lightweight, flexible HTTP client for modern web applications, with support for interceptors, timeouts, retries, and file handling.
Features
🚀
Simple & Intuitive
Easy-to-use API that wraps the native fetch API with powerful features.
⚙️
Request Interceptors
Modify requests before they are sent, perfect for adding authentication headers.
🔄
Response Interceptors
Transform responses before they reach your code, simplifying error handling.
🔁
Automatic Retries
Configurable retry behavior for failed requests with exponential backoff.
📁
File Handling
Built-in support for file uploads and downloads with progress tracking.
📘
TypeScript Support
Full TypeScript support with comprehensive type definitions.
Quick Start
Basic Usage
1// Create a configured instance
2const api = voca.create(fetch, {
3 baseUrl: 'https://api.example.com',
4 timeout: 10000,
5 onResponse: async (response) => {
6 if (!response.ok) {
7 throw new Error(`HTTP Error: ${response.status}`);
8 }
9 return response.json();
10 }
11});
12
13// Make requests with your configured API
14const posts = await api.get('/posts');
15const newPost = await api.post('/posts', { title: 'New Post', body: 'Content' });
File Upload with Progress
1// Upload a file with progress tracking
2const updateProgress = (percent) => {
3 console.log(`Upload progress: ${percent.toFixed(1)}%`);
4 progressBar.style.width = `${percent}%`;
5};
6
7const result = await api.uploadFile(
8 'https://api.example.com/upload',
9 fileObject,
10 { 'Authorization': 'Bearer token' },
11 updateProgress
12);